Typescript/Vue.js
Last modified: May 28 2019 - 1832hrs
Date: May 21 2019 - 1000-1200
Venue: Video Conference Room (VC), COM1 02-13
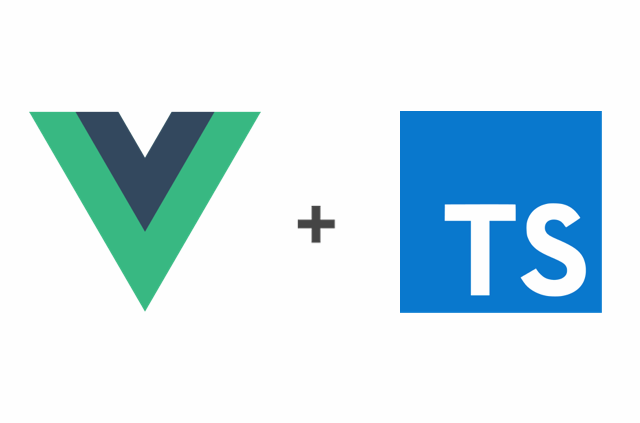
The first part covers typescript in detail
- What problems it solves
- Type definitions
- Modernization
- The new problems it introduces
After that, we go into the details of Vue
- Why modern web frameworks
- Philosophy behind modern frameworks
- Vue itself
- Components
- Data binding
- Reactivity
Readings
Exercises
Assignment
Composition
This assignment involves combining components from the last assignment and doing proper data binding.
Task 0: Look at the webpack.config.js
Setup Vue using a templating system
- I can’t figure out why my webpack is breaking =/
- Stuck for 4+ hours. It’s resolving files in the wrong folder somehow.
Instructions:
- Install vue-cli:
npm install -g @vue/cli-service-global
- Create a project
- typescript, babel, router, linter, css preprocessor
- linter: ts-lint
- css preprocessor: sass/scss
- To run: npm run serve
Task 1: FriendsList
Create a component for the friendslist.
- If done correctly, this involves using the styles from Assignment 2 and converting the template from Assignment 1.
- Load up friends.json in the component and convert it.
Task 2: Messages
Examine services/Message.ts.
- You will randomly receive messages from “friends”.
Complete the Messages component.
Use the vue-router or otherwise to allow selecting the user for which messages should be shown.
Finally, allow sending messages.
- Sent messages should go to messageService.
Task 3: Integrate the layout
Use the layout in Assignment 2 to put the side bar at the side.
- Hint: if you want to know if the menu is out, then
- use v-model to bind the checkbox, this way you know if the checkbox is checked.
Task 4: Creating a Friends Service.
Modify Task 1 to use a Friends service.
- Figure out a better way to write the lastMessage.
- Either
- update Friends service OR
- Encourage the component to use the last message from MessageService.
- Don’t forget to handle the case where the user has no messages.
- Either
Show the user messsages which have not been seen in the FriendsList.
- If the user has not checked on the message in the FriendsList component, then you want to put a style for that.
- In telegram, unseen messages are bold.
- Hint: Please keep to a functional-reactive/declarative style of programming.
Solutions
Check the SOLUTION.md inside.